How do I manually set a cookie in a script?
Simply put, cookies are a way to convey state in the otherwise stateless HTTP protocol. Many sites use them to store and relay information about the user from one request to the next.
Once a cookie is set, the client or browser is expected to send that cookie with every subsequent request to that same server (sometimes only to certain paths).
Cookies are normally set by the Set-Cookie header, but they can also be set by JavaScript. You can read more about the Cookie header on the Mozilla Developer Network.
Setting cookies in protocol scripts
In most cases, it’s not necessary to manually set a Cookie header in a protocol script. Loadster automatically receives Set-Cookie headers from your server and handles the cookies like any other HTTP user agent.
However, if you need to manually pass a cookie from your script that was not received from the server, here is how you would do it.
First of all, click the Add… menu on the step you want to add the cookie for, and choose Add request header.
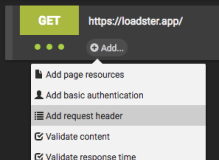
Enter the header name of “Cookie” and the header value as the key-value pair. For this example, we’ll go with a cookie name of “a” and a value of “1”.
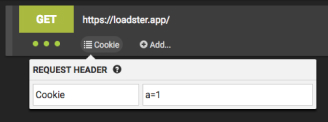
After playing your script, you’ll see a message about the custom request header in the logs:
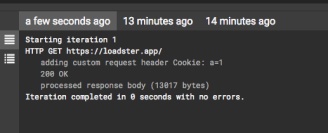
You can also drill into the request to view the exact headers that were sent:
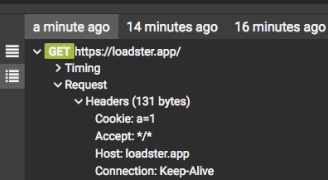
Setting cookies in browser scripts
Browser Bots automate real web browsers. Since the browser handles cookies automatically, and in most cases you don’t need to worry about setting them yourself.
However, there are times you might want to set a cookie manually. For example, you might be testing a site that requires login, but you’re not actually performing the login in the script. Instead you could manually set the cookies that you’d otherwise get by logging in.
Setting cookies in Evaluate Blocks
One way to accomplish this in a browser script is with an evaluate block. Evaluate blocks let you execute custom JavaScript inside the bot’s browser.
An evaluate block that sets a cookie would go something like this:
// Declare a function that sets a cookie
function setCookie(cookieName, cookieValue, expiresInDays) {
const date = new Date();
const expiresInMillis = expiresInDays * 86400000;
date.setTime(date.getTime() + expiresInMillis);
document.cookie = cookieName + '=' + cookieValue + ';expires=' + date.toUTCString() + ';path=/';
}
// Call the function to actually set a cookie
setCookie('myCookieName', 'myCookieValue', 10)
Of course, you can call the function multiple times if you need to set multiple cookies.
Since the JavaScript runs on-page, setting a cookie in an eval block requires that you’ve already navigated to a page, so it won’t work as the first step in your script.
Setting cookies in Code Blocks
You can also set a cookie from a code block.
browser.addCookie({
name: 'myCookieName',
value: 'myCookieValue',
url: 'https://example.com/secure'
});
The cookie will be available to the URL you specify and all sub-URLs.
Since code blocks run outside the bot’s browser, you can use them to set a cookie before navigating to a page.